Chips
Materialize is a modern responsive CSS framework based on Material Design by Google.
Chips can be used to represent small blocks of information. They are most commonly used either for contacts or for tags.
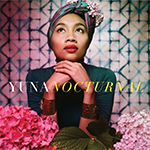
Copied!
content_copy
Jane Doe
Tag
close
check
Filter
close
Information
check
Filter
close
Contacts
To create a contact chip just add an img inside.
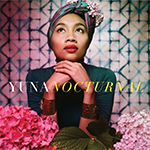
Copied!
content_copy
Jane Doe
Tags
To create a tag chip just add a close icon inside with the class
close
.
Copied!
content_copy
Tag
close
Javascript Plugin
To add tags, just enter your tag text and press enter. You can delete them by clicking on the close icon or by using your delete button.
ATTENTION: Data-Format has changed from version 1.X.X to 2.0.0! Please update option 'data'.
Set initial tags.
Use placeholders and override hint texts.
Use autocomplete with chips.
Copied!
content_copy
Initialization
Copied!
content_copy
document.addEventListener('DOMContentLoaded', function() {
const elems = document.querySelectorAll('.chips');
const instances = M.Chips.init(elems, {
// specify options here
autocompleteOptions: {
data: [
{id: 12, text: "Apple"},
{id: 13, text: "Microsoft"},
{id: 42, text: "Google", image: 'http://placehold.it/250x250'}
]
},
placeholder: 'Enter a tag',
secondaryPlaceholder: '+Tag',
});
});
Chip data object
Copied!
content_copy
const chip = {
id: '4711', // unique identifier (can be a string too)
text: 'Title' // optional Text
image: '', // optional Image-Url
};
Options
Name | Type | Default | Description |
---|---|---|---|
data | Array | [] | Set the chip data (look at the Chip data object) |
placeholder | String | '' | Set first placeholder when there are no tags. |
secondaryPlaceholder | String | '' | Set second placeholder when adding additional tags. |
closeIconClass | String | 'material-icons' | Specifies class to be used in "close" button (useful when working with Material Symbols icon set). |
allowUserInput | Boolean | false | Specifies option to render user input field |
autocompleteOptions | Object | {} | Set autocomplete options. |
autocompleteOnly | Boolean | false | Toggles abililty to add custom value not in autocomplete list. |
limit | Integer | Infinity | Set chips limit. |
onChipAdd | Function | null | Callback for chip add. |
onChipSelect | Function | null | Callback for chip select. |
onChipDelete | Function | null | Callback for chip delete. |
Methods
Use these methods to interact with chips.
All the methods are called on the plugin instance. You can get the plugin instance like this:
Copied! content_copyconst instance = M.Chips.getInstance(elem);
.addChip();
Add chip to input.
Arguments
Chip: Chip data object.
instance.addChip({
id: 1337,
text: 'John Doe', // optional
image: '', // optional
});
.deleteChip();
Delete nth chip.
Arguments
Integer: Index of chip.
instance.deleteChip(3); // Delete 3rd chip.
.selectChip();
Select nth chip.
Arguments
Integer: Index of chip.
instance.selectChip(2); // Select 2nd chip
Properties
Name | Type | Description |
---|---|---|
el | Element | The DOM element the plugin was initialized with. |
options | Object | The options the instance was initialized with. |
chipsData | Array | Array of the current chips data. |
hasAutocomplete | Boolean | If the chips has autocomplete enabled. |
autocomplete | Object | Autocomplete instance, if any. |