Media
Use Box and Slider to present your media content in a cool way.
Media components include things that have to do with large media objects like Images, Video, Audio, etc.
Material Box
Material box is a material design implementation of the Lightbox plugin. When a user clicks on an image that can be enlarged, Material box centers the image and enlarges it in a smooth, non-jarring manner. To dismiss the image, the user can either click on the image again, scroll away, or press the ESC key.
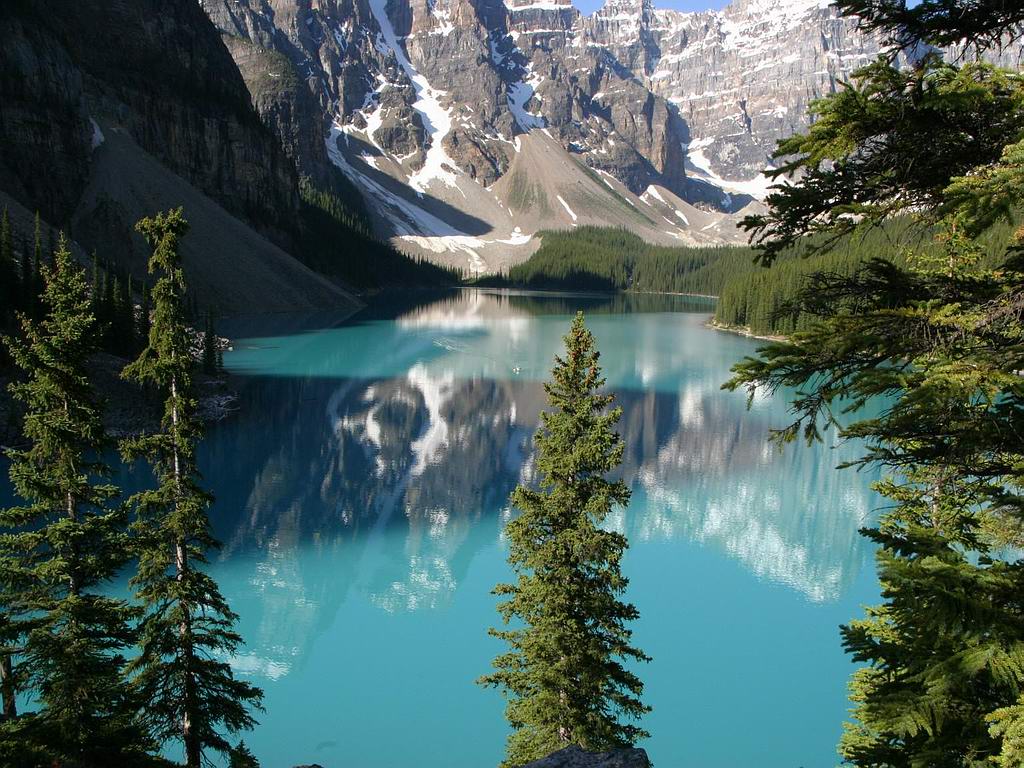
Creating the above image with the effect is as simple as adding a materialboxed
class to the image tag.
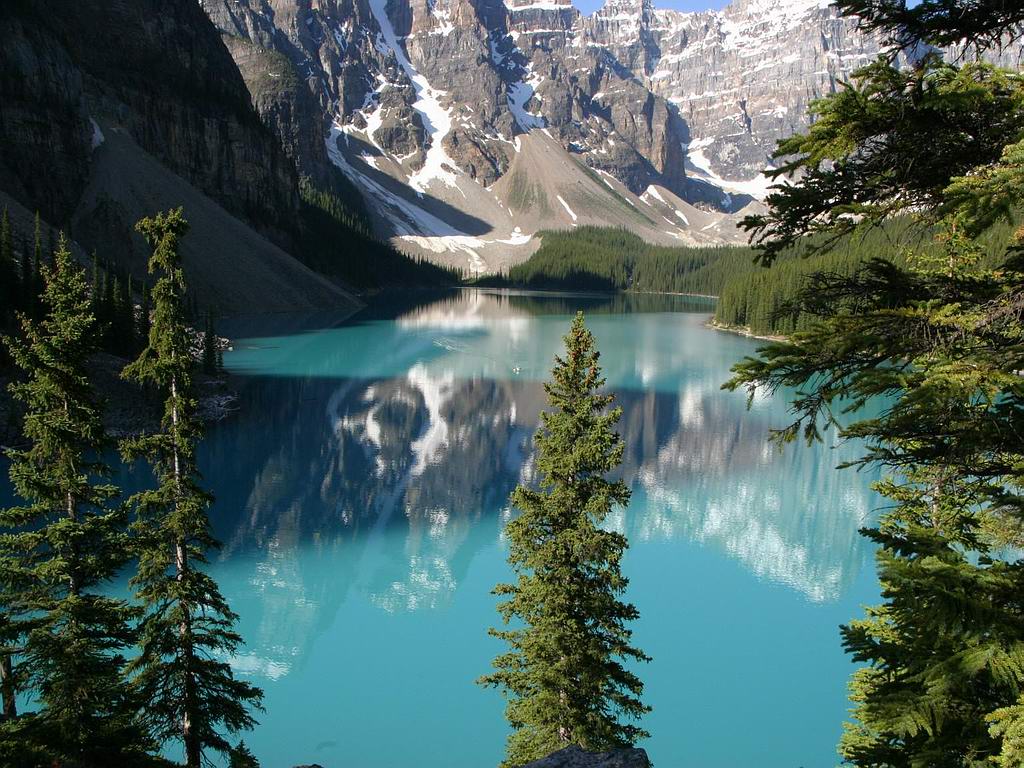
Initialization
document.addEventListener('DOMContentLoaded', function() {
var elems = document.querySelectorAll('.materialboxed');
var instances = M.Materialbox.init(elems, {
// specify options here
});
});
Options
Name | Type | Default | Description |
---|---|---|---|
inDuration | Number | 275 | Transition in duration in milliseconds. |
outDuration | Number | 200 | Transition out duration in milliseconds. |
onOpenStart | Function | null | Callback function called before materialbox is opened. |
onOpenEnd | Function | null | Callback function called after materialbox is opened. |
onCloseStart | Function | null | Callback function called before materialbox is closed. |
onCloseEnd | Function | null | Callback function called after materialbox is closed. |
Methods
All the methods are called on the plugin instance. You can get the plugin instance like this:
var instance = M.Materialbox.getInstance(elem);
.open();
Open materialbox
instance.open();
.close();
Close materialbox
instance.close();
.destroy();
Destroy plugin instance and teardown
instance.destroy();
Properties
Name | Type | Description |
---|---|---|
el | Element | The DOM element the plugin was initialized with. |
options | Object | The options the instance was initialized with. |
overlayActive | Boolean | If the materialbox overlay is showing. |
doneAnimating | Boolean | If the carousel is no longer being animated. |
caption | String | Caption if specified. |
originalWidth | Number | Original width of image. |
originalHeight | Number | Original height of image. |
Captions
It is very easy to add a short caption to your photo. Just add the caption as a
data-caption
attribute.
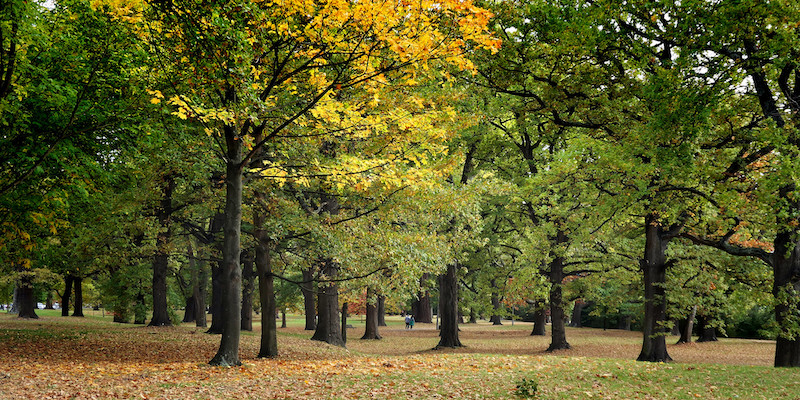
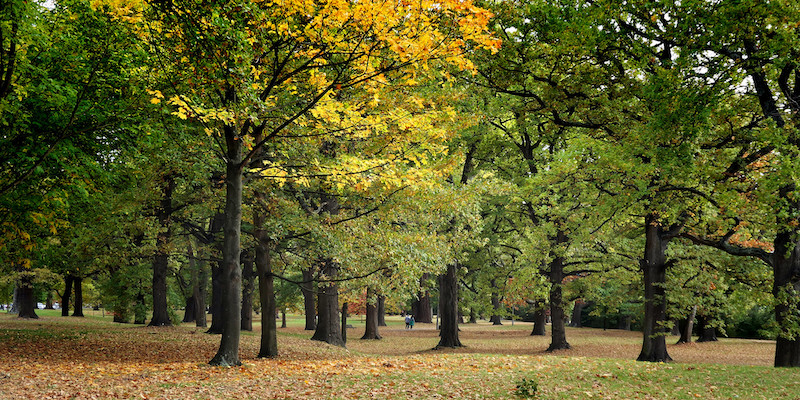
Slider
Our slider is a simple and elegant image carousel. You can also have captions that will be transitioned on their own depending on their alignment. You can also have indicators that show up on the bottom of the slider.
Initialization
document.addEventListener('DOMContentLoaded', function() {
var elems = document.querySelectorAll('.slider');
var instances = M.Slider.init(elems, {
// specify options here
/** Optional function which generates ARIA label for each indicator */
indicatorLabelFunc: (idx, current) => {
let label = "Go to slide " + idx;
if (current) {
label = label + " (Current)";
}
return label;
}
});
});
Options
Name | Type | Default | Description |
---|---|---|---|
indicators | Boolean | true | Set to false to hide slide indicators. |
height | Number | 400 | Set height of slider. |
duration | Number | 500 | Set the duration of the transition animation in ms. |
interval | Number | 6000 | Set the duration between transitions in ms. |
pauseOnFocus | Boolean | true | If slider should pause when keyboard focus is received. |
pauseOnHover | Boolean | true | If slider should pause when is hovered by a pointer. |
indicatorLabelFunc | Function | null |
Optional function used to generate ARIA label to indicators (for accessibility purposes). It receives the current index, starting from "1", and a boolean indicating whether it is the current element or not. If not specified, the generated label is going to be the current index. |
Methods
We have methods to pause, start, move to next and move to previous slide.
All the methods are called on the plugin instance. You can get the plugin instance like this:
var instance = M.Slider.getInstance(elem);
.pause();
Pause slider autoslide
instance.pause();
.start();
Start slider autoslide
instance.start();
.next();
Move to next slider
instance.next();
.prev();
Move to prev slider
instance.prev();
.destroy();
Destroy plugin instance and teardown
instance.destroy();
Properties
Name | Type | Description |
---|---|---|
el | Element | The DOM element the plugin was initialized with. |
options | Object | The options the instance was initialized with. |
activeIndex | Number | Index of current slide. |
eventPause | Boolean | Indicates whether the slider is paused by a focus/mouse event or not. |
Fullscreen Slider
You can easliy make this slider a fullscreen slider by adding the class fullscreen
to the slider div. Here's a quick demo.